Aviary is a very powerful Photo editor. It has tons of filters (like instagram filters) and cool effects and tools (you can crop, change brightness, saturation, tilt shift, add frames, stickers, focus, blur, etc).
They offer all this in a free SDK, which is wrapped in this library. This library is supported by Android 2.3+.
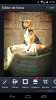
Setup instructions:
1) Sign Up in Aviary: https://developers.aviary.com/signup
2) In your Aviary account add a new app, and take note of the Key and Secret strings.
3) Download the sdk HERE (its too big to upload it here, so I share a Dropbox link). The SDK and the Wrapper are in the "libs" folder, the "res" folder contains resources that you have to add to your projects, which is explained below.
4) Copy all the content of the "libs" folder to your B4A "Additional Libraries" folder.
5) Create a new B4A project, and copy the "res" folder of the sdk to your project folder. So now you will have "Files", "Objects", and "res" folders.
6) In the Project Attributes region add the following line:
7) Now open the Manifest (In B4A: Project -> Manifest Editor), make sure that the minSDKVersion is set to 9 (Android 2.3), and add this lines at the end:
In the line where it says "YOUR_API_KEY" you should change it to the Key you got in 2)
And where it says "YOUR_PACKAGE_NAME" you need to put your actual package name (found in Project -> Package name)
And thats it, here is a little example code. In this example we assume you have an Image you want to edit in your Assets folder, but you can do it with any image (you can choose one from your Albums, or combine it with the output of the camera):
They offer all this in a free SDK, which is wrapped in this library. This library is supported by Android 2.3+.
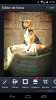
Setup instructions:
1) Sign Up in Aviary: https://developers.aviary.com/signup
2) In your Aviary account add a new app, and take note of the Key and Secret strings.
3) Download the sdk HERE (its too big to upload it here, so I share a Dropbox link). The SDK and the Wrapper are in the "libs" folder, the "res" folder contains resources that you have to add to your projects, which is explained below.
4) Copy all the content of the "libs" folder to your B4A "Additional Libraries" folder.
5) Create a new B4A project, and copy the "res" folder of the sdk to your project folder. So now you will have "Files", "Objects", and "res" folders.
6) In the Project Attributes region add the following line:
Where you should change PATH_TO_YOUR_PROJECT_FOLDER to the corresponding path.#AdditionalRes: PATH_TO_YOUR_PROJECT_FOLDER\res, com.aviary.android.feather
7) Now open the Manifest (In B4A: Project -> Manifest Editor), make sure that the minSDKVersion is set to 9 (Android 2.3), and add this lines at the end:
B4X:
AddApplicationText(
<meta-data
android:name="com.aviary.android.feather.v1.API_KEY"
android:value="YOUR_API_KEY" />
<activity
android:name="com.aviary.android.feather.FeatherActivity"
android:configChanges="orientation|keyboardHidden|screenSize"
android:screenOrientation="unspecified"
android:hardwareAccelerated="true"
android:largeHeap="true"
android:process=":aviarysdk"
android:theme="@style/AviaryTheme" />
<activity
android:name="com.aviary.android.feather.AlertActivity"
android:launchMode="standard"
android:noHistory="true"
android:theme="@style/AviaryTheme.Dialog">
<intent-filter>
<action android:name="aviary.intent.action.ALERT"/>
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
<service
android:process=":aviarycds"
android:name="com.aviary.android.feather.cds.AviaryCdsService"
android:exported="false">
<intent-filter>
<action android:name="aviary.intent.action.CDS_DOWNLOAD_START"/>
<action android:name="aviary.intent.action.CDS_RESTORE_USER_ITEMS"/>
</intent-filter>
</service>
<provider
android:name="com.aviary.android.feather.cds.AviaryCdsProvider"
android:authorities="YOUR_PACKAGE_NAME.AviaryCdsProvider"
android:process=":aviarycds"
android:exported="false"
android:syncable="true" />
<receiver
android:name="com.aviary.android.feather.cds.AviaryCdsReceiver"
android:process=":aviarycds" >
<intent-filter>
<action android:name="android.intent.action.DOWNLOAD_COMPLETE" />
</intent-filter>
</receiver>
)
'End of default text.
And where it says "YOUR_PACKAGE_NAME" you need to put your actual package name (found in Project -> Package name)
And thats it, here is a little example code. In this example we assume you have an Image you want to edit in your Assets folder, but you can do it with any image (you can choose one from your Albums, or combine it with the output of the camera):
B4X:
Sub Globals
Dim AviaryActivity As Aviary
Dim BtnStart As Button
End Sub
Sub Activity_Create(FirstTime As Boolean)
BtnStart.Initialize("BtnStart")
BtnStart.Text = "Start Aviary!"
Activity.AddView(BtnStart,25%x,100%y - 64dip, 50%x, 48dip)
'First we copy the image in the Assets folder to an other folder, so that we can pass it as parameter to Aviary
If Not(File.Exists(File.DirDefaultExternal,"MyImage.jpg")) Then
File.Copy(File.DirAssets,"MyImage.jpg",File.DirDefaultExternal,"MyImage.jpg")
End If
If FirstTime Then
AviaryActivity.Initialize(File.Combine(File.DirDefaultExternal,"MyImage.jpg"),APP_SECRET)
AviaryActivity.SetOutputFile(File.Combine(File.DirDefaultExternal,"MyImageWithAviary.jpg"))
End If
End Sub
Sub Aviary_Result(IsSuccess As Boolean)
'Do whatever you want with the processed Image now, for example show it in a imageview:
Log(IsSuccess)
If (IsSuccess) Then
Dim Bmp As Bitmap
Dim AspectRatio As Float
Dim Iv As ImageView
Bmp.Initialize(File.DirDefaultExternal,"MyImageWithAviary.jpg")
AspectRatio = Bmp.Width/Bmp.Height
Iv.Initialize("")
Iv.Gravity = Gravity.FILL
Iv.Bitmap = Bmp
Activity.AddView(Iv,10%x,20%y,80%x,80%x/AspectRatio)
End If
End Sub
Sub BtnStart_Click
AviaryActivity.StartAviary
End Sub
Last edited: