A BLE connection is made between a central device and a peripheral device. In most cases the Android device will be the central device.
Beacons, heart rate sensors and other BLE devices are implemented as peripheral device.
However the Android device can also implement the peripheral role. This is done with this library.
It is supported by Android 5.0 (API 21+). Not all devices support this feature.
Unofficial list of devices that support peripheral mode: https://altbeacon.github.io/android-beacon-library/beacon-transmitter-devices.html
This library is implemented in a similar way to B4i peripheral feature: https://www.b4x.com/android/forum/threads/ble-chat-connecting-android-and-ios.66543/
It creates a service (UUID = 0001) with two characteristics:
ReadChar (1001) - Other devices should subscribe for notifications on this characteristics.
WriteChar (1002) - Other devices can write to this characteristic.
The NewData event is raised when a device writes to WriteChar.
Other devices receive a notification when you call Peripheral.Write.
Setup steps
It is always recommended to implement communication related code in a service. The Starter service is a good place.
1. Initialize a BleManager2 object.
2. The StateChanged event will be raised. Assuming that the state is STATE_POWERED_ON you should initialize the BlePeripheral2 object.
3. Make sure that peripheral role is supported with the IsPeripheralSupported property.
4. Call Start to start advertising.
The Subscribe event will be raised when a central device connects and registers for notifications.
Multiple central devices can subscribe to a single peripheral.
Example of a chat implementation with Android and iOS devices is attached.
Updates
- B4A projects were updated with targetSdkVersion set to 26 and the coarse permission is requested in the central project.
- BLE2Peripheral v1.12 is attached. It allows overriding the default advertising settings. Example:
https://developer.android.com/reference/android/bluetooth/le/AdvertiseSettings.Builder
Beacons, heart rate sensors and other BLE devices are implemented as peripheral device.
However the Android device can also implement the peripheral role. This is done with this library.
It is supported by Android 5.0 (API 21+). Not all devices support this feature.
Unofficial list of devices that support peripheral mode: https://altbeacon.github.io/android-beacon-library/beacon-transmitter-devices.html
This library is implemented in a similar way to B4i peripheral feature: https://www.b4x.com/android/forum/threads/ble-chat-connecting-android-and-ios.66543/
It creates a service (UUID = 0001) with two characteristics:
ReadChar (1001) - Other devices should subscribe for notifications on this characteristics.
WriteChar (1002) - Other devices can write to this characteristic.
The NewData event is raised when a device writes to WriteChar.
Other devices receive a notification when you call Peripheral.Write.
Setup steps
It is always recommended to implement communication related code in a service. The Starter service is a good place.
1. Initialize a BleManager2 object.
2. The StateChanged event will be raised. Assuming that the state is STATE_POWERED_ON you should initialize the BlePeripheral2 object.
3. Make sure that peripheral role is supported with the IsPeripheralSupported property.
4. Call Start to start advertising.
The Subscribe event will be raised when a central device connects and registers for notifications.
Multiple central devices can subscribe to a single peripheral.
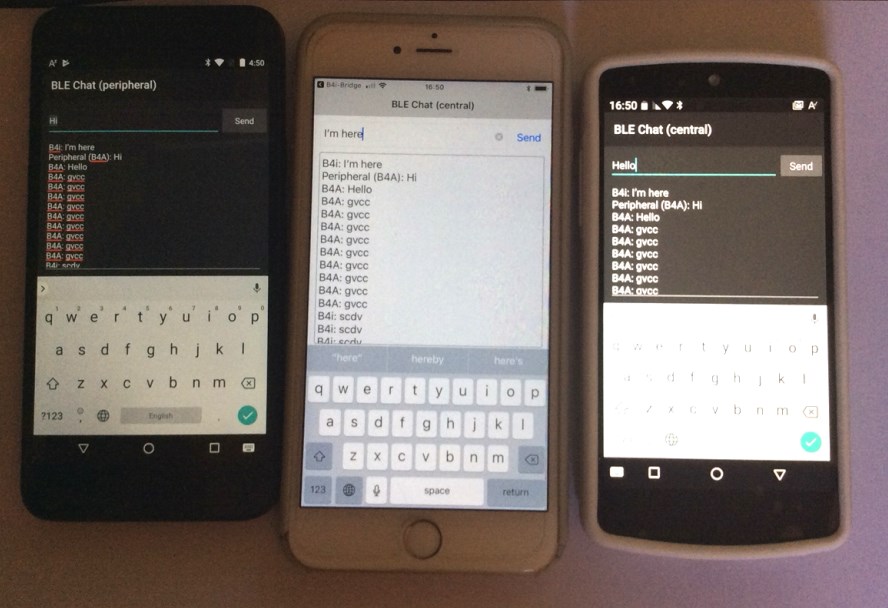
Example of a chat implementation with Android and iOS devices is attached.
Updates
- B4A projects were updated with targetSdkVersion set to 26 and the coarse permission is requested in the central project.
- BLE2Peripheral v1.12 is attached. It allows overriding the default advertising settings. Example:
B4X:
peripheral.Start2("B4APeripheral", CreateAdvertiseSettings)
Private Sub CreateAdvertiseSettings As Object
Dim builder As JavaObject
builder.InitializeNewInstance("android.bluetooth.le.AdvertiseSettings.Builder", Null)
builder.RunMethod("setConnectable", Array(True))
builder.RunMethod("setAdvertiseMode", Array(2)) 'ADVERTISE_MODE_LOW_LATENCY
builder.RunMethod("setTxPowerLevel", Array(3)) 'ADVERTISE_TX_POWER_HIGH
Return builder.RunMethod("build", Null)
End Sub
Attachments
Last edited: