SQL Injection is a common vulnerability, where a specifically crafted user input is executed by the SQL engine. It is one of the most common vulnerabilities.
Example of vulnerable code that checks the user password:
Dim count As Int = SQL.ExecuteQuerySingleResult("SELECT count(*) WHERE password = '" & EditText1.Text & '")
If count > 0 Then
Log("Welcome authenticated user!")
End If
A user can enter: ‘ or ‘a’ = ‘a. This results in the following WHERE clause: password = ” or ‘a’ = ‘a’. It will match all records.
Another problem with this code is that the code will break if the input includes an apostrophe.
As the solution is so simple, there is really no excuse to write such code. Correct code:
Dim count As Int = SQL.ExecuteQuerySingleResult2("SELECT count(*) WHERE password = ?", Array As String(EditText1.Text))
If count > 0 Then
Log("Welcome authenticated user!")
End If
The SQL engine will treat the user input as a single parameter value. No need to escape anything and no need to worry from SQL injections.
Simple and safe.
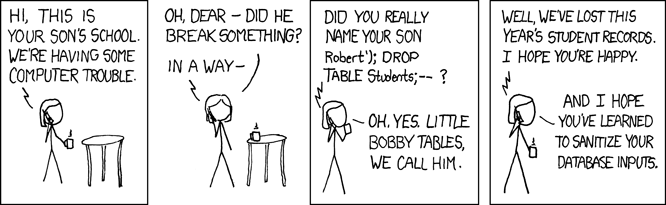