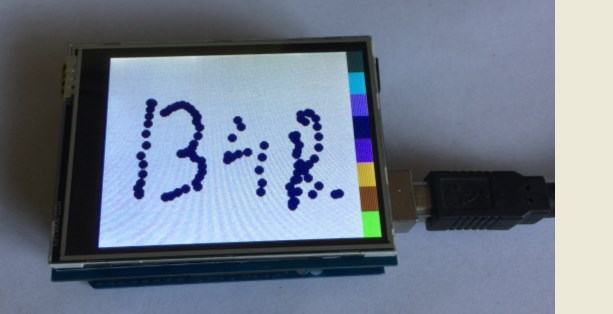
Libraries for Adafruit TFT screen: https://www.adafruit.com/products/1651
https://learn.adafruit.com/adafruit-2-8-tft-touch-shield-v2
rAdafruit_STMPE610 handles the touch events.
rAdafruit_ILI9341 handles the graphics. It depends on rAdafruitGFX library.
Example code (depends on rAdafruit_STMPE610, rAdafruit_ILI9341 and rAdafruitGFX libraries):
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private STMP As AdafruitSTMPE610
Private ILI As AdafruitILI93411
Private colors(8) As UInt
Private currentColor As UInt
Private colorsHeight As UInt = 20
Private colorsWidth As UInt
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
If STMP.Initialize(8, "STMP_Touch") = False Then
Log("Failed to initialize touch screen.")
Return
End If
ILI.Initialize(10, 9)
ILI.FillScreen(ILI.COLOR_WHITE)
ILI.GFX.ConfigureText(2, ILI.COLOR_BLACK, True)
ILI.GFX.SetCursor(2, 2)
ILI.GFX.DrawText("This is a test")
Dim p As Pin
p.Initialize(0, p.MODE_INPUT)
RndSeed(p.AnalogRead)
colorsWidth = ILI.GFX.Width / colors.Length
For i = 0 To colors.Length - 1
colors(i) = ILI.Color(Rnd(0, 256), Rnd(0, 256), Rnd(0, 256))
ILI.GFX.DrawRect(colorsWidth * i, 0, colorsWidth, colorsHeight, colors(i), True)
Next
currentColor = colors(0)
End Sub
Sub STMP_Touch (X As Int, Y As Int)
'map the touch range to the drawing screen range
Dim fx As Int = MapRange(X, 150, 3800, 0, ILI.GFX.Width)
Dim fy As Int = MapRange(Y, 130, 4000, 0, ILI.GFX.Height)
If fy < colorsHeight Then
currentColor = colors(fx / colorsWidth)
ILI.GFX.DrawRect(0, ILI.GFX.Height - 3, ILI.GFX.Width, ILI.GFX.Height, currentColor, True)
Log("CurrentColor: ", currentColor)
Else
ILI.GFX.DrawCircle(fx, fy, 5, currentColor,True)
End If
End Sub