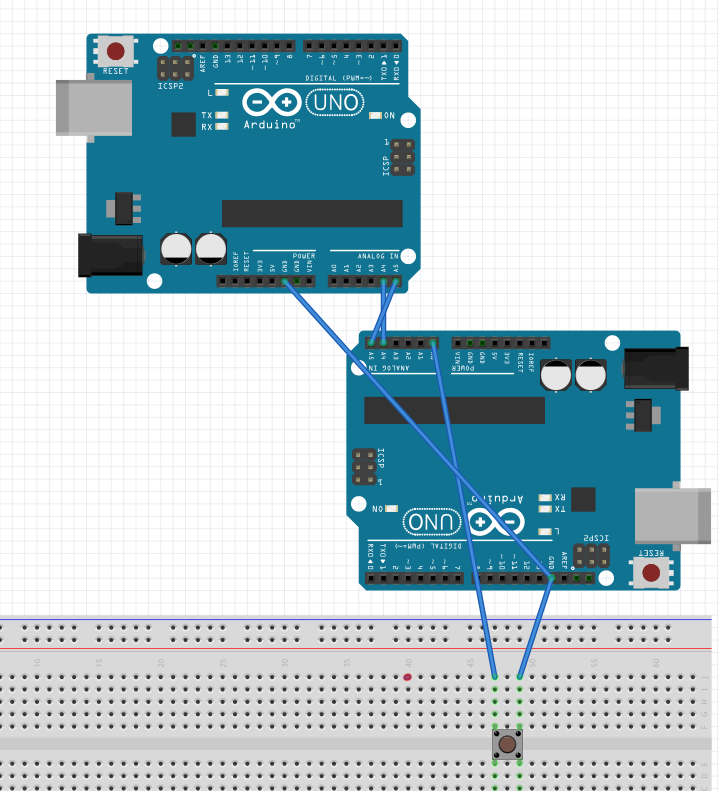
The rWire supports communication with other I2C/TWI devices.
One device acts as the master and the other devices act as slaves.
Each slave has a unique address between 8 to 127.
You can see the relevant pins here: https://www.arduino.cc/en/Reference/Wire
The master can send data to any slave. The slaves prepare data for the master.
In this example we connect two Arduinos. The master will read the state of the the button which is connected to the slave board.
You can run two instances of B4R. One for the slave and one for the master.
This is the slave code:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private slave As WireSlave
Private btn As Pin
Private const SLAVE_ADDRESS As Byte = 8
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
slave.Initialize(SLAVE_ADDRESS, "Slave_NewData")
btn.Initialize(btn.A0, btn.MODE_INPUT_PULLUP)
btn.AddListener("Btn_StateChanged")
End Sub
Sub Btn_StateChanged (State As Boolean)
Dim b(1) As Byte
'It will be False when button is down
If State Then b(0) = 0 Else b(0) = 1
slave.SetDataForMaster(b) '<-- it will only be read when the master calls RequestFrom
End Sub
Sub Slave_NewData (Data() As Byte)
Log("Received from master: ", Data)
End Sub
The master code:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private timer1 As Timer
Private master As WireMaster
Private const SLAVE_ADDRESS As Byte = 8
Private led13 As Pin
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
master.Initialize
led13.Initialize(13, led13.MODE_OUTPUT)
led13.DigitalWrite(True)
timer1.Initialize("Timer1_Tick", 10)
timer1.Enabled = True
End Sub
Sub Timer1_Tick
master.WriteTo(SLAVE_ADDRESS, NumberFormat(Millis, 0, 0).GetBytes)
Dim b() As Byte = master.RequestFrom(SLAVE_ADDRESS, 1)
If b.Length = 1 Then
Dim state As Boolean
If b(0) = 0 Then state = False Else state = True
led13.DigitalWrite(state)
End If
End Sub