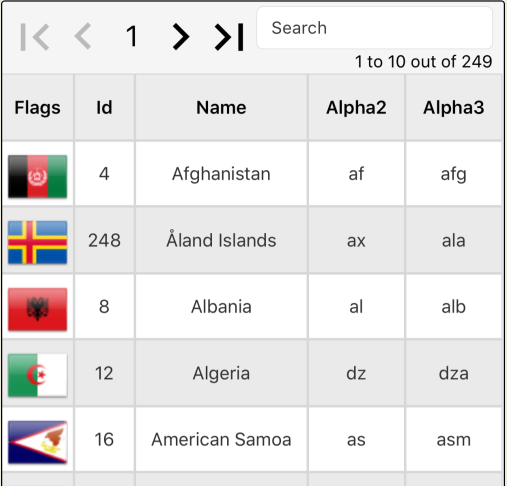
Requires B4XTable v1.01+: https://www.b4x.com/android/forum/t...searchable-customizable-table.102322/#content
This example demonstrates the steps required to create cells with custom layouts.
Each cell in B4XTable is made of a Panel with a Label. The cells layouts are created as needed when more rows become visible. The cells are reused when the user switches to a different page (or whenever the data is updated).
To make it simpler to use custom layouts we start with:
B4X:
B4XTable1.MaximumRowsPerPage = 10
B4XTable1.BuildLayoutsCache(10)
The purpose of these two lines is to limit the maximum number of rows per page and to force B4XTable to create all the cells. This way we don't need to deal with cases where more rows become visible. For example when the form is resized in B4J.
Next step is to create the custom layout. In this case we want to add an ImageView to each of the flags column cells.
B4X:
For i = 1 To FlagsColumn.CellsLayouts.Size - 1
Dim pnl As B4XView = FlagsColumn.CellsLayouts.Get(i)
Dim iv As ImageView
iv.Initialize("")
pnl.AddView(iv, 5dip, 5dip, FlagsColumn.Width - 10dip, B4XTable1.RowHeight - 10dip)
Next
The last step is to update the flags whenever the visible data changes:
B4X:
Sub B4XTable1_DataUpdated
For i = 0 To B4XTable1.VisibleRowIds.Size - 1
Dim RowId As Long = B4XTable1.VisibleRowIds.Get(i)
Dim pnl As B4XView = FlagsColumn.CellsLayouts.Get(i + 1) '+1 because the first cell is the header
Dim iv As B4XView = pnl.GetView(1) 'ImageView will be the 2nd child of the panel. The built-in label is the first.
If RowId > 0 Then
Dim row As Map = B4XTable1.GetRow(RowId)
iv.SetBitmap(xui.LoadBitmapResize(File.DirAssets, row.Get("Alpha2") & ".png", iv.Width, iv.Height, True))
Else
'empty row
iv.SetBitmap(Null)
End If
Next
End Sub
The RowId of empty rows is set to 0. As the cells are reused we need to remove the bitmap.
B4A example is attached. The same code will work in B4J and B4i as well.
The flags and countries data source: https://github.com/stefangabos/world_countries/