New method to add shortcuts: https://www.b4x.com/android/forum/threads/support-for-app-shortcuts.86663/#post-548645
Updated instructions for this method: https://www.b4x.com/android/forum/threads/solved-shortcut-example-not-working.105195/#post-659178
Android allows the user to add desktop shortcuts to any application.
Pressing on such a shortcut is exactly the same as starting your application from the applications list.
However it is also possible to create custom shortcuts which will have a custom behavior. For example start a different activity or start your application in a different mode.
In order to add a custom shortcut you should create a dedicated "shortcut Activity". This activity will be started when the user adds your shortcut from the shortcuts list. The activity should return an intent as the result which will later start the application.
The following screenshots demonstrate the shortcut behavior on a Honeycomb device. It should be similar to regular devices:
Shortcuts list:
Desktop:
Our application launched from the shortcut:
You should edit the manifest file and set an intent filter to the shortcut activity. This tells the OS that your application includes a custom activity.
Please read the section about manual modification of the manifest file here.
This is the shortcut activity declaration in the manifest file of the example program:
android:name is the lower cased name of the shortcut activity.
android:label is the title that will appear in the shortcuts list.
The code of the shortcut activity is:
You should change the line with the comment to match your package name and target activity. Note that the syntax is: package_name/.activity and activity should be lower cased.
The last step that should be done is to check in our main activity whether our program was launched from the shortcut:
Updated instructions for this method: https://www.b4x.com/android/forum/threads/solved-shortcut-example-not-working.105195/#post-659178
Android allows the user to add desktop shortcuts to any application.
Pressing on such a shortcut is exactly the same as starting your application from the applications list.
However it is also possible to create custom shortcuts which will have a custom behavior. For example start a different activity or start your application in a different mode.
In order to add a custom shortcut you should create a dedicated "shortcut Activity". This activity will be started when the user adds your shortcut from the shortcuts list. The activity should return an intent as the result which will later start the application.
The following screenshots demonstrate the shortcut behavior on a Honeycomb device. It should be similar to regular devices:
Shortcuts list:
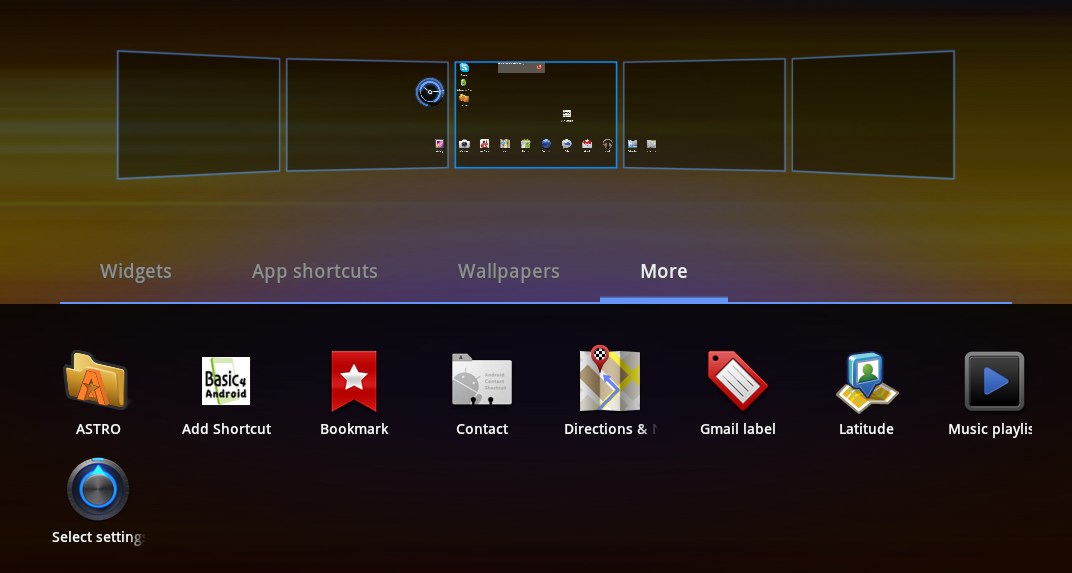
Desktop:
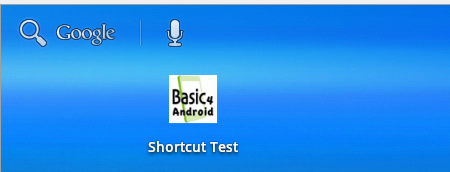
Our application launched from the shortcut:
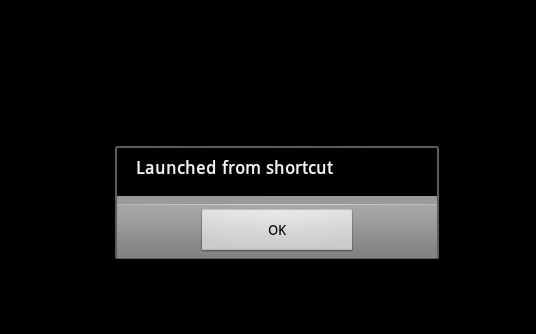
You should edit the manifest file and set an intent filter to the shortcut activity. This tells the OS that your application includes a custom activity.
Please read the section about manual modification of the manifest file here.
This is the shortcut activity declaration in the manifest file of the example program:
B4X:
<activity android:windowSoftInputMode="stateHidden" android:launchMode="singleTop" android:name="shortcutactivity"
android:label="Add Shortcut" android:screenOrientation="unspecified">
<intent-filter>
<action android:name="android.intent.action.CREATE_SHORTCUT"/>
<category android:name="android.intent.category.DEFAULT"/>
</intent-filter>
</activity>
android:label is the title that will appear in the shortcuts list.
The code of the shortcut activity is:
B4X:
Sub Activity_Create(FirstTime As Boolean)
Dim shortcutIntent As Intent
shortcutIntent.Initialize("", "")
shortcutIntent.SetComponent("anywheresoftware.b4a.samples.test/.main") '<--- change to match your package name / and target activity.
shortcutIntent.PutExtra("from_shortcut", True)
Dim in As Intent
in.Initialize("", "")
in.PutExtra("android.intent.extra.shortcut.INTENT", shortcutIntent)
in.PutExtra("android.intent.extra.shortcut.NAME", "Shortcut Test")
in.PutExtra("android.intent.extra.shortcut.ICON", LoadBitmap(File.DirAssets, "small_logo.png"))
Activity.SetActivityResult(-1, in)
Activity.Finish
End Sub
The last step that should be done is to check in our main activity whether our program was launched from the shortcut:
B4X:
Sub Activity_Resume
Dim in As Intent
in = Activity.GetStartingIntent
Log(in)
If in.HasExtra("from_shortcut") AND in.GetExtra("from_shortcut") = True Then
Msgbox("Launched from shortcut", "")
End If
End Sub
Attachments
Last edited: