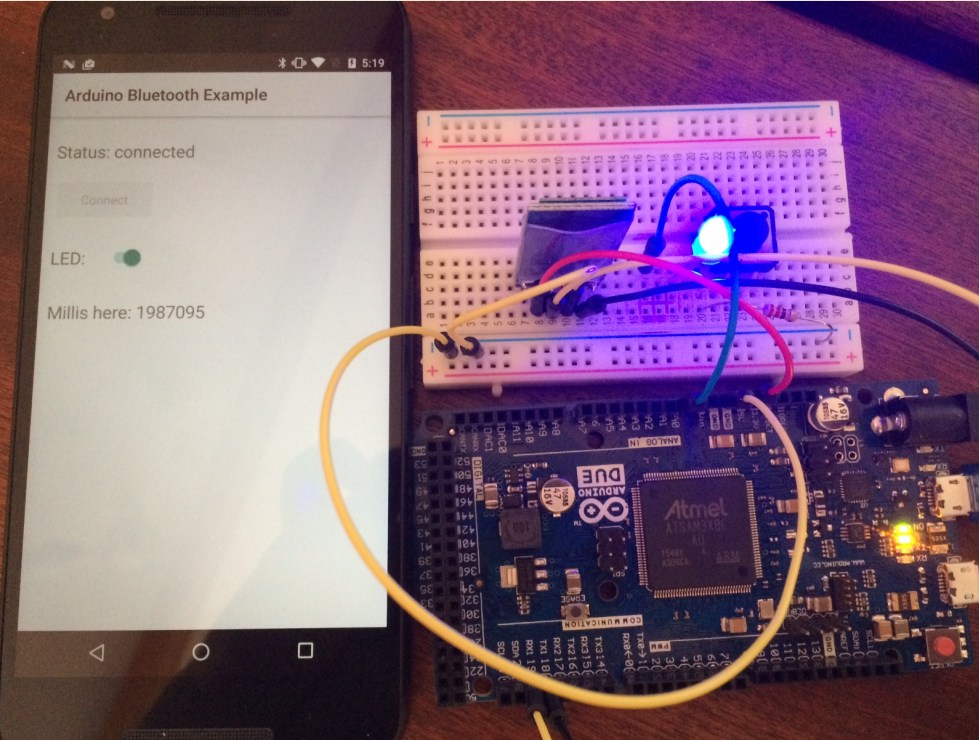
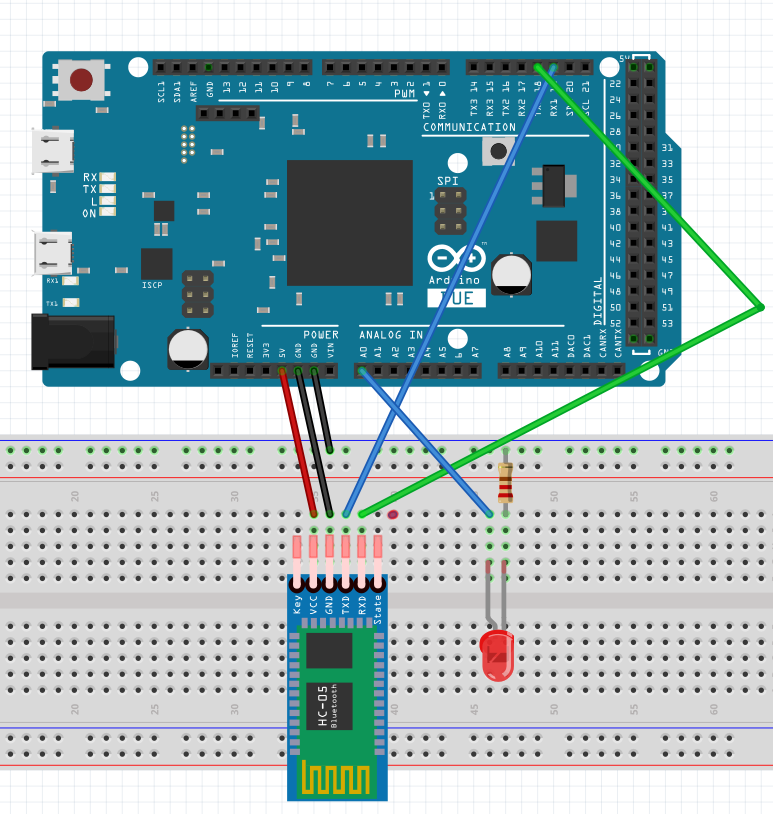
In this example we will connect an Android device to an Arduino using class Bluetooth with the HC 05 module.
Working with this module is simple. Connect the power and gnd pins and connect the HC05 RX pin to an Arduino TX pin and the HC05 TX pin to an Arduino RX pin.
The HC05 RX pin expects 3.3 volt. In this case I'm using the Arduino Due which is a 3.3v board. However most boards are 5v so you will need to add two resistors as explained here: http://www.martyncurrey.com/arduino-with-hc-05-bluetooth-module-in-slave-mode/
You can use the rSoftwareSerial library to turn two regular pins to a serial port. If you are using a board such as Mega or Due then you can use one of the additional hardware serial ports.
This is done with the following code:
B4X:
RunNative("SerialNative1", Null)
#if C
void SerialNative1(B4R::Object* unused) {
::Serial1.begin(9600);
b4r_main::_serialnative1->wrappedStream = &::Serial1;
}
#end if
The Arduino will send the current millis value every second. It will send it as a string. In most cases it is simpler and better to work with binary data instead of strings. However for this example I chose to send a string and to read it in the Android side with AsyncStreamsText. AsyncStreamsText takes care of collecting the received data and parsing it based on the end of line characters.
The Android will send commands to turn on or off the led(s).
B4X:
'b4r
Sub AStream_NewData (Buffer() As Byte)
For i = 0 To Buffer.Length - 2 Step 2
Dim ledNumber As Byte = Buffer(i)
Dim value As Boolean = Buffer(i + 1) = 1
leds(ledNumber).DigitalWrite(value)
Next
End Sub
'b4a
Sub Switch1_CheckedChange(Checked As Boolean)
Dim b As Byte
If Checked Then b = 1 Else b = 0
CallSub2(Starter, "SendMessage", Array As Byte(0, b))
End Sub
Complete B4R code:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private SerialNative1 As Stream
Private astream As AsyncStreams
Private leds(1) As Pin
Private timer1 As Timer
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
leds(0).Initialize(leds(0).A0, leds(0).MODE_OUTPUT)
'Using the hardware serial named Serial1 (Arduino Due)
'A SoftwareSerial will also work.
RunNative("SerialNative1", Null)
astream.Initialize(SerialNative1, "astream_NewData", Null)
timer1.Initialize("timer1_Tick", 1000)
timer1.Enabled = True
End Sub
#if C
void SerialNative1(B4R::Object* unused) {
::Serial1.begin(9600);
b4r_main::_serialnative1->wrappedStream = &::Serial1;
}
#end if
Sub Timer1_Tick
astream.Write("Millis here: ".GetBytes)
astream.Write(NumberFormat(Millis, 0, 0).GetBytes)
astream.Write(Array As Byte(10)) 'end of line character. AsyncStreamsText will cut the message here
End Sub
Sub AStream_NewData (Buffer() As Byte)
For i = 0 To Buffer.Length - 2 Step 2
Dim ledNumber As Byte = Buffer(i)
Dim value As Boolean = Buffer(i + 1) = 1
leds(ledNumber).DigitalWrite(value)
Next
End Sub
Attachments
Last edited: