This is an example of how UDP can be used to broadcast the current temperature and pressure to one or more clients.
The nice thing about it is that there is no server involved. The ESP8266 just sends the packets to the broadcast address.
RandomAccessFile is used to create the packets. B4R doubles are 4 bytes (equivalent to B4X floats).
It is tempting to send the data as a string. However it is more efficient and actually simpler to deal with binary encoded numeric values.
This is the main code of this example (B4R):
The clients code:
The broadcast address is usually the network address with 255 as the fourth byte. The B4J program prints it.
BMP180 library is available here: https://www.b4x.com/android/forum/threads/bmp180-pressure-and-temperature.67730/
The nice thing about it is that there is no server involved. The ESP8266 just sends the packets to the broadcast address.
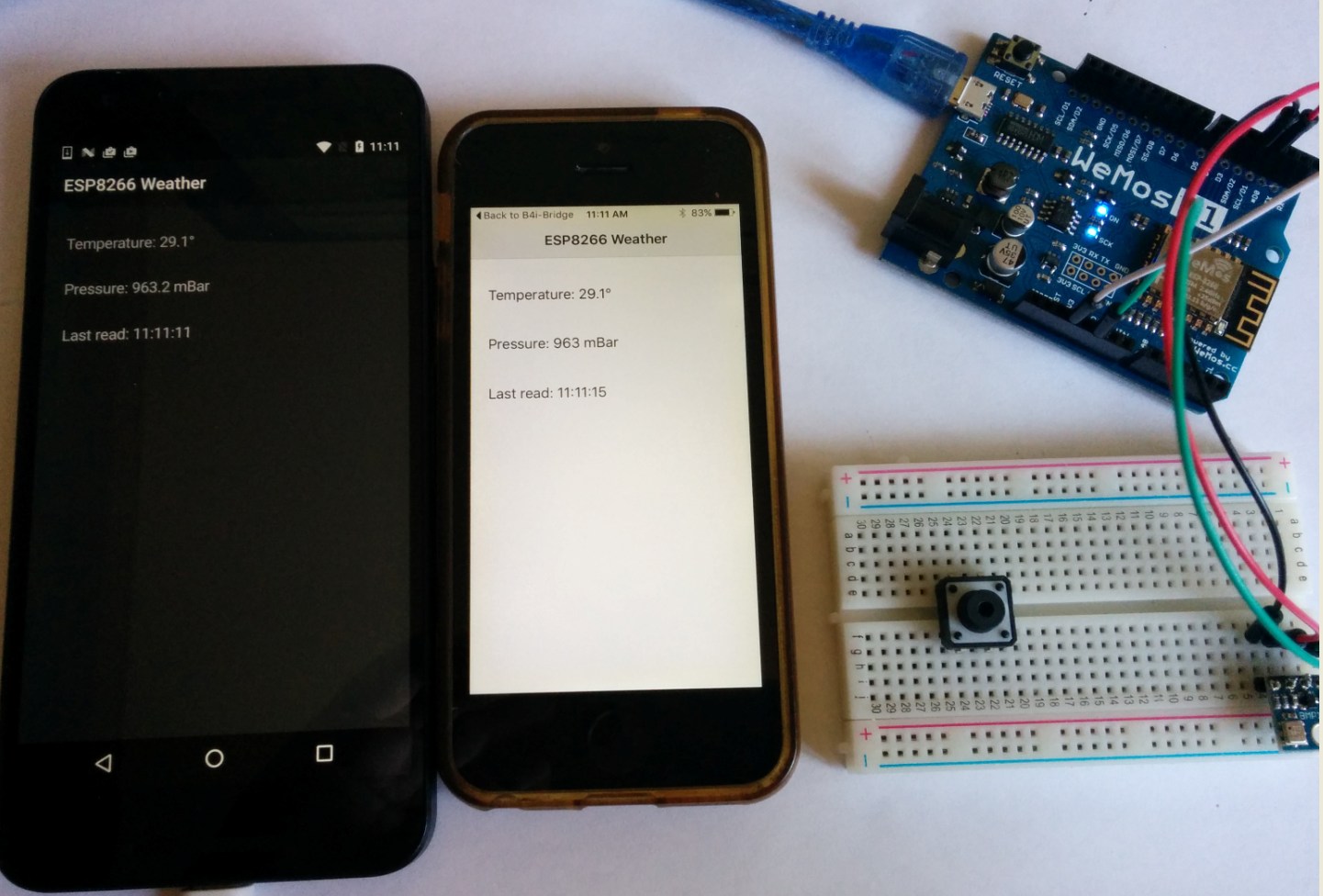
RandomAccessFile is used to create the packets. B4R doubles are 4 bytes (equivalent to B4X floats).
It is tempting to send the data as a string. However it is more efficient and actually simpler to deal with binary encoded numeric values.
This is the main code of this example (B4R):
B4X:
Dim buffer(8) As Byte
Dim raf As RandomAccessFile
raf.Initialize(buffer, True)
raf.WriteDouble32(bmp180.LastResult, raf.CurrentPosition)
bmp180.GetPressure(0, bmp180.LastResult)
raf.WriteDouble32(bmp180.LastResult, raf.CurrentPosition)
usocket.BeginPacket(ip, port)
usocket.Write(buffer)
usocket.SendPacket
The clients code:
B4X:
Private Sub usocket_PacketArrived (Packet As UDPPacket)
If Packet.Length <> 8 Then
Log("Invalid data")
Return
Else
Dim raf As RandomAccessFile
raf.Initialize3(Packet.Data, True)
raf.CurrentPosition = Packet.Offset
Dim temperature, pressure As Float
temperature = raf.ReadFloat(raf.CurrentPosition)
pressure = raf.ReadFloat(raf.CurrentPosition)
Log($"Temperature: $1.1{temperature}°"$)
Log($"Pressure: $1.1{pressure} mBar"$)
End If
End Sub
The broadcast address is usually the network address with 255 as the fourth byte. The B4J program prints it.
BMP180 library is available here: https://www.b4x.com/android/forum/threads/bmp180-pressure-and-temperature.67730/