Arduino Uno with LCD shield:
Working with a LCD monitor:
1. Initialize the LiquidCrystal object and set the connected pins.
2. Call Begin with the number of columns and rows:
3. Call Write to print to the LCD. The message can be made of a string, a number or an array of bytes.
You can call Write multiple times.
This example is a bit more complicated.
It works together with the "external serial connector": https://www.b4x.com/android/forum/threads/tool-external-serial-connector.65724/ (a B4J desktop program).
Once the PC is connected to the Arduino, the PC sends the current time (there is no clock on the Arduino).
B4R code:
The Arduino starts a timer that shows the current time:
The PC can be disconnected afterwards.
The serial connector program used is slightly modified. It sends the time with this code:
It calls this sub 5 seconds after the connection with the help of CallSubPlus.
The two projects are attached.
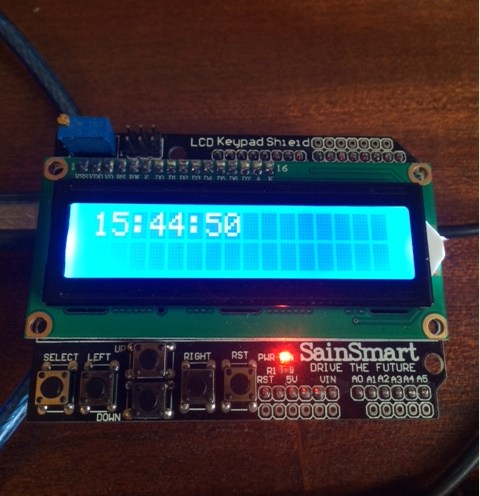
Working with a LCD monitor:
1. Initialize the LiquidCrystal object and set the connected pins.
B4X:
lcd.Initialize(8, 255, 9, Array As Byte (4, 5, 6, 7))
B4X:
lcd.Begin(16, 2)
You can call Write multiple times.
This example is a bit more complicated.
It works together with the "external serial connector": https://www.b4x.com/android/forum/threads/tool-external-serial-connector.65724/ (a B4J desktop program).
Once the PC is connected to the Arduino, the PC sends the current time (there is no clock on the Arduino).
B4R code:
B4X:
Sub AStream_NewData (Buffer() As Byte)
If Buffer.Length <> 3 Then
Log("invalid data: ", Buffer)
Else
hours = Buffer(0)
minutes = Buffer(1)
seconds = Buffer(2)
lcd.Clear
timer1.Enabled = True
Log("B4R: Received time data!")
End If
End Sub
The Arduino starts a timer that shows the current time:
B4X:
Sub Timer1_Tick
seconds = seconds + 1
If seconds = 60 Then
seconds = 0
minutes = minutes + 1
End If
If minutes = 60 Then
minutes = 0
hours = hours + 1
End If
If hours = 24 Then hours = 0
lcd.SetCursor(0, 0)
lcd.Write(NumberFormat(hours, 2, 0))
lcd.Write(":")
lcd.Write(NumberFormat(minutes, 2, 0))
lcd.Write(":")
lcd.Write(NumberFormat(seconds, 2, 0))
End Sub
The PC can be disconnected afterwards.
The serial connector program used is slightly modified. It sends the time with this code:
B4X:
Sub Send_Time
Dim now As Long = DateTime.Now
astream.Write(Array As Byte(DateTime.GetHour(now), DateTime.GetMinute(now), DateTime.GetSecond(now)))
End Sub
The two projects are attached.