I've been working on this project for a long time and I'm very proud to release the version 4 today.
The UltimateListView is, as its pompous name says, THE ListView.
All texts and images can be loaded asynchronously (from Internet, from a database or from a local folder), so you can scroll even if the data are not fully loaded.
The list has its own state manager.
Since September 2018, ULV is available for free. You can still donate for it if you wish.
To send the money, just click on the Donate button below (the amount to enter is in euros):
Note that UltimateListView is not a wrapper around the work of someone else. It is 100% my own code and it is based upon the standard Java ListView of Android.
The UltimateListView does not work with Android versions < 2. It cannot work with B4J or B4i.
Current version: 4.50
DOWNLOAD HERE:
The UltimateListView is, as its pompous name says, THE ListView.
- It can handle very long lists. This is a screenshot of a list with 245813 items, all different:
- It can mix different layouts (and they can be changed dynamically). You can use it as an expandable ListView:
- It has a low memory footprint and is very fast (this report comes from the Performance demo where the list has to display 128901 distinct words read from a database and the used device is a Huawei Honor single core 1.4 Ghz):
- It can scroll in both directions thanks to its swipe detector:
- The swipe detector can also be used to implement a swipe-to-dismiss or a swipe-to-reveal:
- You can easily add editors to your table to change its content:
- You can animate the items when they are added, removed, replaced or when the list is scrolled (with your own custom animation):
- It can stack items from the bottom:
- It supports drag & drop operations (internal & external):
- You can synchronize lists with different item heights:
All texts and images can be loaded asynchronously (from Internet, from a database or from a local folder), so you can scroll even if the data are not fully loaded.
The list has its own state manager.
Since September 2018, ULV is available for free. You can still donate for it if you wish.
To send the money, just click on the Donate button below (the amount to enter is in euros):
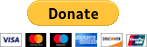
Note that UltimateListView is not a wrapper around the work of someone else. It is 100% my own code and it is based upon the standard Java ListView of Android.
The UltimateListView does not work with Android versions < 2. It cannot work with B4J or B4i.
Current version: 4.50
DOWNLOAD HERE:
Last edited: