Very simple to use Bluetooth library for ESP32 modules.
Note that you need to have a recent version of ESP32 SDK. If you encounter any error then download the complete SDK: https://github.com/espressif/arduino-esp32 and copy it to ESP32 SDK folder:
C:\Users\<user>\Documents\Arduino\hardware\espressif\esp32
Instructions:
1. Initialize a ESP32Bluetooth object. You pass the name and the StateChanged event sub. It returns True if it was successful.
2. Initialize an AsyncStreams object with bt.Stream. Based on my tests the connection is not 100% stable when you send a lot of data. It is therefore recommended to use non-prefix mode.
3. The StateChanged event will be raised whenever the connection state changes. AStreams_Error will never be raised.
Example of Bluetooth chat with an Android device:
The B4A project is attached.
B4R code:
Note that you need to have a recent version of ESP32 SDK. If you encounter any error then download the complete SDK: https://github.com/espressif/arduino-esp32 and copy it to ESP32 SDK folder:
C:\Users\<user>\Documents\Arduino\hardware\espressif\esp32
Instructions:
1. Initialize a ESP32Bluetooth object. You pass the name and the StateChanged event sub. It returns True if it was successful.
2. Initialize an AsyncStreams object with bt.Stream. Based on my tests the connection is not 100% stable when you send a lot of data. It is therefore recommended to use non-prefix mode.
3. The StateChanged event will be raised whenever the connection state changes. AStreams_Error will never be raised.
Example of Bluetooth chat with an Android device:
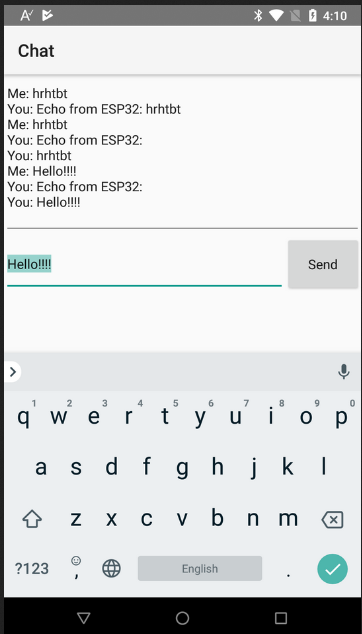
The B4A project is attached.
B4R code:
B4X:
#Region Project Attributes
#AutoFlushLogs: True
#CheckArrayBounds: True
#StackBufferSize: 600
#End Region
Sub Process_Globals
Public Serial1 As Serial
Private bt As ESP32Bluetooth
Private astream As AsyncStreams
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
If bt.Initialize("Test1", "bt_StateChanged") = False Then
Log("Failed to start Bluetooth")
Return
End If
astream.Initialize(bt.Stream, "astream_NewData", "astream_Error")
End Sub
Sub bt_StateChanged (Connected As Boolean)
Log("connected: ", Connected)
End Sub
Sub AStream_Error
Log("error")
End Sub
Sub AStream_NewData (Buffer() As Byte)
Log("NewData: ", Buffer)
astream.Write("Echo from ESP32: ")
astream.Write(Buffer)
bt.Stream.Flush
End Sub