rEthernet library v1.10 (included in v1.00 beta 9) includes a new type named EthernetUDP. It allows sending and receiving UDP packets.
UDP is simple to work with as it is a connection-less protocol.
Steps required:
1. Initialize an Ethernet object with a static ip address or InitializeDHCP.
2. Initialize the EthernetUDP object and set the port number.
3. The PacketArrived event will be raised when a packet is received.
4. Sending packets:
Example of communication between an Android an Arduino:
B4R program:
The B4A program is attached.
UDP is simple to work with as it is a connection-less protocol.
Steps required:
1. Initialize an Ethernet object with a static ip address or InitializeDHCP.
2. Initialize the EthernetUDP object and set the port number.
3. The PacketArrived event will be raised when a packet is received.
4. Sending packets:
B4X:
udp.BeginPacket(serverIp, serverPort) '<--- Begin
udp.Write("Button is ".GetBytes) '<-- One or more calls to Write
If State = False Then
udp.Write("down".GetBytes)
Else
udp.Write("up".GetBytes)
End If
udp.SendPacket '<--- Send
Example of communication between an Android an Arduino:
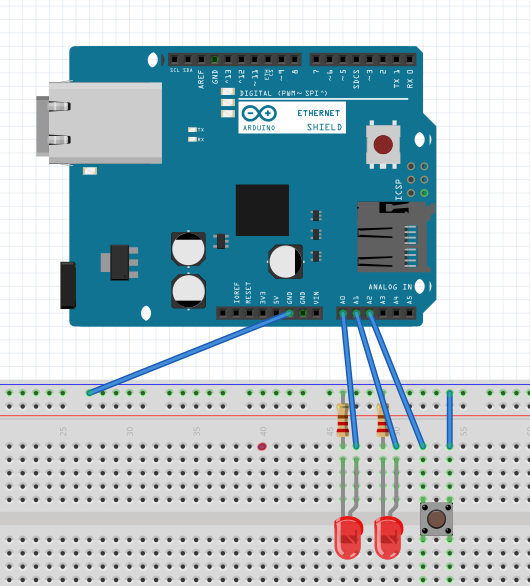
B4R program:
B4X:
#Region Project Attributes
#AutoFlushLogs: True
#StackBufferSize: 300
#End Region
Sub Process_Globals
Public Serial1 As Serial
Private eth As Ethernet
Private udp As EthernetUDP
Private serverIp() As Byte = Array As Byte(192, 168, 0, 13)
Private MacAddress() As Byte = Array As Byte(0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED)
Private const serverPort As UInt = 51042
Private leds(2) As Pin
Private btn As Pin
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
leds(0).Initialize(leds(0).A0, leds(0).MODE_OUTPUT)
leds(1).Initialize(leds(0).A1, leds(0).MODE_OUTPUT)
btn.Initialize(btn.A2, btn.MODE_INPUT_PULLUP)
btn.AddListener("btn_StateChanged")
If eth.InitializeDHCP(MacAddress) = False Then
Log("Error connecting to network.")
Return
Else
Log("Connected to network. My ip address: ", eth.LocalIp)
End If
udp.Initialize(12345, "udp_PacketArrived")
End Sub
Sub Udp_PacketArrived (Data() As Byte, Ip() As Byte, Port As UInt)
Log("PacketArrived from:")
PrintIp(Ip)
If Data.Length < 2 Then Return
'first byte is the led index
'second byte is the state
leds(Data(0)).DigitalWrite(Data(1) = 1)
End Sub
Sub PrintIp(ip() As Byte)
Log(ip(0),".", ip(1),".", ip(2),".", ip(3))
End Sub
Sub Btn_StateChanged (State As Boolean)
udp.BeginPacket(serverIp, serverPort)
'(It makes more sense to send a single byte instead of a string.)
udp.Write("Button is ".GetBytes)
If State = False Then
udp.Write("down".GetBytes)
Else
udp.Write("up".GetBytes)
End If
Log(udp.SendPacket)
End Sub
The B4A program is attached.