Updated Oct 30, 2023
Attached is a small project (version 19) to get a grip on Google Drive.Most work was done by mw71, but this brings it all together from scattered informations to a usable entrypoint.
' ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~--' Previous Info sources on B4X (sorted by date DEC)' ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~--' 2018-06-01, Erel, OAUTH2, v2.10 --> https://www.b4x.com/android/forum/threads/class-b4x-google-oauth2.79426/#content' 2017-08-26, mw71, testconnect, --> https://www.b4x.com/android/forum/threads/problems-with-oauth.80174/page-2#post-526872'' 2017-08-18, mw71, googledrive_playing --> https://www.b4x.com/android/forum/threads/problems-with-oauth.80174/#post-524978' 2017-06-20, mw71, Testconnect --> https://www.b4x.com/android/forum/threads/googledrive-via-api-v3.80775/#post-512176' 2017-06-18, mw71, GDrive via APIv3 --> https://www.b4x.com/android/forum/threads/googledrive-via-api-v3.80775/' 2017-06-04, mw71, upload file --> https://www.b4x.com/android/forum/threads/http-patch-request.80261/#post-508444' ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~-- ~~~--
' 2023-10-28, JohnC, changes in libs and modules --> https://www.b4x.com/android/forum/t...st-api-v3-small-testproject.95778/post-964342
Libraries:
Modules:
Manifest:
B4X:
'This code will be applied to the manifest file during compilation.
'You do not need to modify it in most cases.
'See this link for for more information: https://www.b4x.com/forum/showthread.php?p=78136
AddManifestText(
<uses-sdk android:minSdkVersion="20" android:targetSdkVersion="33"/>
<supports-screens android:largeScreens="true"
android:normalScreens="true"
android:smallScreens="true"
android:anyDensity="true"/>)
SetApplicationAttribute(android:icon, "@drawable/icon")
SetApplicationAttribute(android:label, "$LABEL$")
'End of default text.
AddActivityText(Main,
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="$PACKAGE$" />
</intent-filter>
)
Notes:
1. The module GoogleOauth2 contains "Sub Testconnect" from mw71
2. Read and understand the project specific setup below
Create a new project in the Google AP developer console
Select "Google Drive API" in the dashboard
Enable the API
Create credentials
As alerted by (1) on first entry you may create the credentials via button (2) right now. It is recommended to create credentials via button (3)
You need to fill in "SHA-1" and "Package name". Both are available in your B4A IDE:
Then receive your client ID:
The program sequence is as follows:
1. "GoogleDrive" is initialized in Main with the "ClientId" for Oauth2
1.1 "GoogleDrive" initializes "GoogleOauth2" and informs about the "Scope= ....drive"
2. Main calls "ConnectToDrive" and waits for message from "GD_Connected".
2.1 "GoogleDrive" requests an AccessToken via "GoogleOauth2"
2.2 "GoogleDrive" executes a "TestConnect" and reports via "GD_Connected" to waiting Main
3. Main displays the AccessToken in Label1
With the AccessToken several actions can be can performed with the drive:
If you need a "ClientSecret" at some point create a new OAuth2 ClientId with the ApplicationType "Web application"
B4X:
' ------------------------------------------------------------------------------
Sub btnStart_Click As ResumableSub
Log("#-btnStart_Click -----------------------------------")
Label1.Text = "(Trying to connect to Google Drive...)"
GD.Initialize(Me, "GD", ClientIdOauth, ClientSecret) ', AppApiKey)
GD.ConnectToDrive
wait for GD_Connected(mapRet As Map)
' ww~~-- ww~~-- ────────────────────
'
'Log("#- x129, GD_Connected, mapRet=" & mapToPrettyString(mapRet) )
Label1.Text = "Access_token= " & mapRet.GetDefault("access_token", "?")
'
GD.ShowFileList("")
wait for GD_FileListResult(lstFiles As List)
' ww~~-- ww~~-- ────────────────────
'
Log("#-GD_FileListResult, lstFiles=" & lstToPrettyString(lstFiles) )
ListView1.Clear
Dim lblX As Label
lblX = ListView1.SingleLineLayout.Label
lblX.TextSize = 12
lblX.TextColor = Colors.Green
For i=0 To lstFiles.Size -1
ListView1.AddSingleLine(lstFiles.Get(i))
Next
'
btnCreaFolder.Enabled = True
btnUpload.Enabled = True
btnDownload.Enabled = True
btnSearch.Enabled = True
'
Return Null
End Sub
' ------------------------------------------------------------------------------
Sub btnCreaFolder_Click As ResumableSub
Log("#-")
Log("#- ---*** ---*** ---*** ---*** ---*** ---*** ---*** ---*** ")
Log("#-Sub btnCreaFolder_Click")
Dim strFolderParentID As String = ""
edtFolderToCreate.Text = "Testfolder_01"
Label3.Text = $"(Trying to create folder ${edtFolderToCreate.Text})"$
GD.CreateFolder(edtFolderToCreate.Text, strFolderParentID)
wait for GD_FolderCreated(strFileId As String)
' ww~~-- ww~~-- ────────────────────
'
Label3.Text = "FileId= " & strFileId
Log("#- x178, strFileId=" & strFileId)
Return Null
End Sub
' ------------------------------------------------------------------------------
Sub btnUpload_Click As ResumableSub
Log("#-")
Log("#- ---*** ---*** ---*** ---*** ---*** ---*** ---*** ---*** ")
Log("#-Sub btnUpload_Click")
Dim strFolderParentID As String = ""
Dim strFilenameInGdrive As String = "testdatafile4"
EditText2.Text = strFilenameInGdrive
Dim strFileToUpload As String = "testdata2.json"
Label2.Text = $"(Trying to upload ${strFileToUpload})"$
GD.UploadFile("", File.DirAssets, strFileToUpload, strFolderParentID, strFilenameInGdrive)
wait for GD_FileUploadDone(strFileId As String)
' ww~~-- ww~~-- ────────────────────
'
Label2.Text = "FileId= " & strFileId
Log("#- x130, strFileId=" & strFileId)
edtFileToDownload.Text = strFileId
Return Null
End Sub
' ------------------------------------------------------------------------------
Sub btnSearch_Click As ResumableSub
Log("#-")
Log("#- ---*** ---*** ---*** ---*** ---*** ---*** ---*** ---*** ")
Log("#-Sub btnSearch_Click")
Label4.Text = $"(Trying to search for ${EditText2.Text.Trim})"$
GD.SearchForFileID(EditText2.Text.Trim, EditText1.Text.Trim)
wait for GD_FileFound(strFileId As String)
' ww~~-- ww~~-- ────────────────────
'
Label4.Text = "FileId= " & strFileId
Log("#- x170, strFileId=" & strFileId)
Return Null
End Sub
' ------------------------------------------------------------------------------
Sub btnDownload_Click As ResumableSub
Log("#-")
Log("#- ---*** ---*** ---*** ---*** ---*** ---*** ---*** ---*** ")
Log("#-Sub btnDownload_Click")
Label5.Text = $"(Trying to download ${edtFileToDownload.Text.Trim})"$
GD.DownloadFile(edtFileToDownload.Text.Trim, File.DirDefaultExternal, "aaa_file_" & edtFileToDownload.Text.Trim)
wait for GD_FileDownloaded(strRet As String)
' ww~~-- ww~~-- ────────────────────
'
Label5.Text = "strRet= " & strRet
Log("#- x203, strRet=" & strRet)
End Sub
' ------------------------------------------------------------------------------
During the first run you might see a warning if the app hasn't been verified: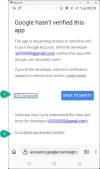
Just click "Advanced" and "Go to grive..."
Now you're ready to test:
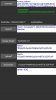
- Google Drive basics of files and folders
- Professional Blog (some specific to C#, but the mechanism explanations are easy to understand)
- Some GoogleDrive error hints
B4X:
' Sub ConnectToDrive
' Sub ShowFileList(ParentFolderID As String)
' Sub CreateFolder(FolderName As String, ParentFolderID As String)
' Sub UploadFile(FileId As String, LocalPath As String, LocalFilename As String, ParentFolderID As String, Name As String)
' Sub DownloadFile(FileID As String, LocalPath As String, LocalFilename As String)
' Sub SearchForFileID(SearchFile As String, ParentFolderID As String)
' Sub SearchForFolderID(SearchFolder As String, ParentFolderID As String)
If something is fundamentally wrong, please explain and enclose a corresponding test project.
Questions should be asked in the Android questions forum including errormessages or code in code-tags.
Edit: Testproject updated to 19 due to module, lib and setupcondition bits.
Info: If you have trouble with apostrophes in the name of files or folders then this will help (thanks to @Dave O): https://www.b4x.com/android/forum/t...api-for-folder-names-with-apostrophes.130495/
Attachments
Last edited: