The EEPROM is in most cases the only persistent storage available. As a persistent storage we can use it to store data that will not be lost when the board is turned off.
The rEEPROM library allows us to read and write from the EEPROM.
This example shows how to read and write from the EEPROM with ByteConverter object.
We will count the number of times that the program has started.
Make sure to first check the two libraries: rRandomAccessFile and rEEPROM:
The program flow is:
- Check whether the "special mark" is stored in bytes 0 - 1.
bc.IntsFromBytes(b)(0) converts the array of bytes to an array of Ints (each int is made of two bytes) and then returns the first, and only, int from the array.
- If not then this is the first time that our program is running. Store the special mark and set the counter to 0.
- If we did find the special mark then read the current counter value from bytes 2 - 3.
- The last step is to increase the counter and write it to bytes 2 - 3:
The complete code:
Run it and press on the reset button:
The rEEPROM library allows us to read and write from the EEPROM.
This example shows how to read and write from the EEPROM with ByteConverter object.
We will count the number of times that the program has started.
Make sure to first check the two libraries: rRandomAccessFile and rEEPROM:
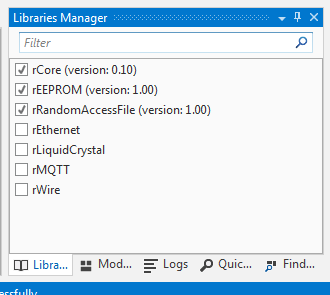
The program flow is:
- Check whether the "special mark" is stored in bytes 0 - 1.
B4X:
'bc is a ByteConverter object.
Dim b() As Byte = eeprom.ReadBytes(0, 2)
If bc.IntsFromBytes(b)(0) = SpecialMark Then
- If not then this is the first time that our program is running. Store the special mark and set the counter to 0.
B4X:
eeprom.WriteBytes(bc.IntsToBytes(Array As Int(SpecialMark)), 0)
counter = 0
B4X:
b = eeprom.ReadBytes(2, 2)
counter = bc.IntsFromBytes(b)(0)
- The last step is to increase the counter and write it to bytes 2 - 3:
B4X:
counter = counter + 1
Log("counter: ", counter)
'write the value to the EEPROM.
eeprom.WriteBytes(bc.IntsToBytes(Array As Int(counter)), 2)
The complete code:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private eeprom As EEPROM
Private bc As ByteConverter
Private const SpecialMark As Int = 12345
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
'without this delay we will miss the first run as the program is reset when the logger
'is connected.
Delay(3000)
Log("AppStart")
'the special mark is stored in bytes 0, 1
'the counter is stored in bytes 2,3
'check if this is the first time
Dim counter As Int
Dim b() As Byte = eeprom.ReadBytes(0, 2)
If bc.IntsFromBytes(b)(0) = SpecialMark Then
'not first time
b = eeprom.ReadBytes(2, 2)
counter = bc.IntsFromBytes(b)(0)
Else
'write the special mark
Log("first time")
eeprom.WriteBytes(bc.IntsToBytes(Array As Int(SpecialMark)), 0)
counter = 0
End If
counter = counter + 1
Log("counter: ", counter)
'write the value to the EEPROM.
eeprom.WriteBytes(bc.IntsToBytes(Array As Int(counter)), 2)
End Sub
Run it and press on the reset button:
B4X:
AppStart
first time
counter: 1
AppStart
counter: 2
AppStart
counter: 3
AppStart
counter: 4
AppStart
counter: 5
AppStart
counter: 6
AppStart
counter: 7
AppStart
counter: 8