Lists together with custom types make a powerful combination:
A custom type defines a simple object with all kinds of fields. (It is a bit like a class without code, though it can do things that cannot be done with classes.)
Instead of making a list with the values directly we now make a list with more information.
First we define the custom type, this is done in Process_Globals:
Each item stores a value, a name and a color.
We can let the IDE generate a Create sub:
There is nothing special about the generated sub. You can modify it as you need.
Adding elements using the CreatePieItem sub:
Now when we iterate over the items we iterate over PieItems:
This allows us to later draw the items names:
Code: (don't forget to add the Type declaration to Process_Globals)
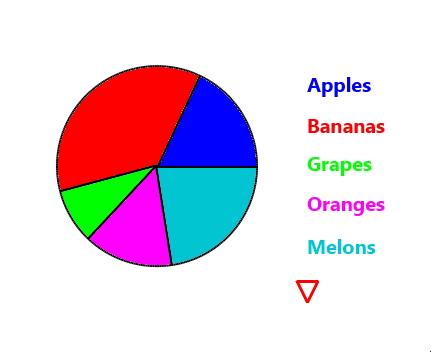
A custom type defines a simple object with all kinds of fields. (It is a bit like a class without code, though it can do things that cannot be done with classes.)
Instead of making a list with the values directly we now make a list with more information.
First we define the custom type, this is done in Process_Globals:
B4X:
Type PieItem (Value As Float, Name As String, Clr As Int)
We can let the IDE generate a Create sub:
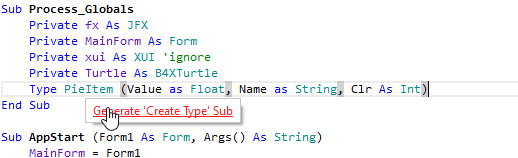
There is nothing special about the generated sub. You can modify it as you need.
Adding elements using the CreatePieItem sub:
B4X:
Items.Add(CreatePieItem(100, "Apples", xui.Color_Blue))
Items.Add(CreatePieItem(200, "Bananas", xui.Color_Red))
Items.Add(CreatePieItem(50, "Grapes", xui.Color_Green))
Now when we iterate over the items we iterate over PieItems:
B4X:
For Each pi As PieItem In Items
TotalValues = TotalValues + pi.value
Next
B4X:
For Each pi As PieItem In Items
Turtle.SetPenColor(pi.Clr).DrawText(pi.Name).MoveForward(40)
Next
Code: (don't forget to add the Type declaration to Process_Globals)
B4X:
Sub Turtle_Start
Turtle.SetSpeedFactor(3).SetPenColor(xui.Color_Black)
Turtle.SetX(Turtle.Width / 2 - 80)
Dim Items As List
Items.Initialize
Items.Add(CreatePieItem(100, "Apples", xui.Color_Blue))
Items.Add(CreatePieItem(200, "Bananas", xui.Color_Red))
Items.Add(CreatePieItem(50, "Grapes", xui.Color_Green))
Items.Add(CreatePieItem(80, "Oranges", xui.Color_Magenta))
Items.Add(CreatePieItem(120, "Melons", 0xFF00C5D0))
DrawPie(Items)
DrawLabels(Items)
End Sub
Sub DrawLabels (Items As List)
Turtle.PenUp.MoveForward(150)
Turtle.SetFontAndAlignment(xui.CreateDefaultBoldFont(20), "LEFT")
Turtle.SetAngle(90).MoveBackward(80)
For Each pi As PieItem In Items
Turtle.SetPenColor(pi.Clr).DrawText(pi.Name).MoveForward(40)
Next
Turtle.PenDown
End Sub
Sub DrawPie (Items As List)
Dim radius As Float = 100
Dim TotalValues As Float
'For Each - best option when we don't need the index
For Each pi As PieItem In Items
TotalValues = TotalValues + pi.value
Next
Dim TotalAngles As Float
'regular For Next
For i = 0 To Items.Size - 1 'first time index is always 0
Dim pi As PieItem = Items.Get(i)
Dim angle As Float = Floor(pi.value / TotalValues * 360)
If i = Items.Size - 1 Then
'to avoid rounding errors the last element completes the circle.
angle = Ceil(360 - TotalAngles)
End If
TotalAngles = TotalAngles + angle
DrawSlice(angle, radius, pi.Clr)
Next
End Sub
Sub DrawSlice (angle As Float, radius As Float, clr As Int)
Turtle.MoveForward(radius).TurnLeft(90)
For a = 1 To angle
Turtle.MoveForward(2 * cPI * radius / 360).TurnLeft(1)
Next
Turtle.TurnLeft(90).MoveForward(100).TurnLeft(180).PenUp
Turtle.TurnRight(angle / 2).MoveForward(radius / 2).SetPenColor(clr).Fill
Turtle.SetPenColor(xui.Color_Black).MoveBackward(radius / 2).TurnLeft(angle / 2).PenDown
End Sub
Public Sub CreatePieItem (Value As Float, Name As String, Clr As Int) As PieItem
Dim t1 As PieItem
t1.Initialize
t1.Value = Value
t1.Name = Name
t1.Clr = Clr
Return t1
End Sub